In this article, we will learn what is REPL in node js and why we use REPL in node js.
What is REPL in node js?
REPL is a very useful feature of Node js. It is used to Experiment of Node js code and Debug the Node js Code
READ:- Read Given input by the user, and store it in memory
EVAL:- Takes and Evaluate the Data Structure
PRINT:- Print the Result or Output
LOOP:- Loops above command until the user presses ctlr+c twice
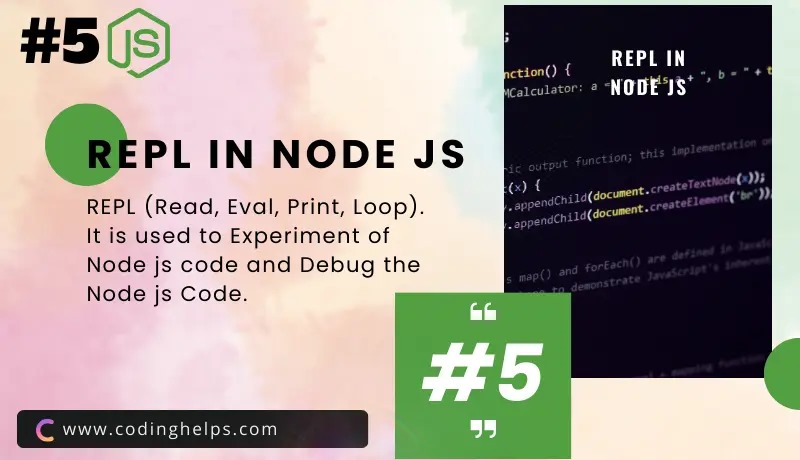
How to use REPL in VS Code Editor
create a new folder and open a new folder in the VS code editor. After That open terminal (ctrl + ~ ).
Type node and enter. now you can use REPL. type .help and show basic command of REPL
PS E:\Practice> node Welcome to Node.js v18.12.1. Type ".help" for more information. > .help .break Sometimes you get stuck, this gets you out .clear Alias for .break .editor Enter editor mode .exit Exit the REPL .help Print this help message .load Load JS from a file into the REPL session .save Save all evaluated commands in this REPL session to a file Press Ctrl+C to abort current expression, Ctrl+D to exit the REPL >
Getting Started With REPL in Node js
we will execute some different types of tasks using REPL
1) Js Expression using REPL
in REPL, we can do anything arithmetic operation like addition, subtraction, multiplication, division, and all other mathematical operations.
Addition
PS E:\Practice> node Welcome to Node.js v18.12.1. Type ".help" for more information. > 3+4 7 > 9+12 21 >
subtraction
PS E:\Practice> node Welcome to Node.js v18.12.1. Type ".help" for more information. > 55-32 23 > 30-20 10 >
using the below example we can do anything operation in the REPL
Also Read this :- prerequisites of node js
2) Use Variable
We can use variables in REPL as we use variables in JavaScript. we can perform String operations and other operations.
In REPL, variables are used for storing value at specific locations with user-friendly name
PS E:\Practice> node Welcome to Node.js v18.12.1. Type ".help" for more information. > > var a = "Coding " undefined > var b = "Helps" undefined > a + b 'Coding Helps' > var a = 30 undefined > var b = 40 undefined > b - a 10 >
3) multiline code
we can also write multiple line codes in REPL. we can create functions, like:- UDF (user defined function), loops, and any other block of codes
in REPL, we can also create loops and a whole program of JavaScript.
PS E:\Practice> node Welcome to Node.js v18.12.1. Type ".help" for more information. > for(i=1; i<5; i++){ ... console.log(`value of i ${i}`); ... } value of i 1 value of i 2 value of i 3 value of i 4 undefined >
4) use underscore ( _ ) to get the last operation result
when we work with REPL then if we want to use previous result data so we can use underscore ( _ ) for getting the last operation result data.
example: 20 is the answer of the last operation and we want to use the last result for the next operation and then write > _ + 10
PS E:\Practice> node Welcome to Node.js v18.12.1. Type ".help" for more information. > 17 + 3 20 > _ + 10 30 > _ - 16 14 >
5) Editor Mode
Editor mode is the same as multiline code. when we open editor mode then we can write multiple line code like we can create functions and blocks of code
write .editor for enter in editor mode. and press Ctrl + D for terminate editor mode
PS E:\Practice> node Welcome to Node.js v18.12.1. Type ".help" for more information. > .editor // Entering editor mode (Ctrl+D to finish, Ctrl+C to cancel) const myfunc = (myname) => { console.log(`My name is ${myname}`)} myfunc("coding helps") My name is coding helps undefined >